Make app listen only after DB connection in Node
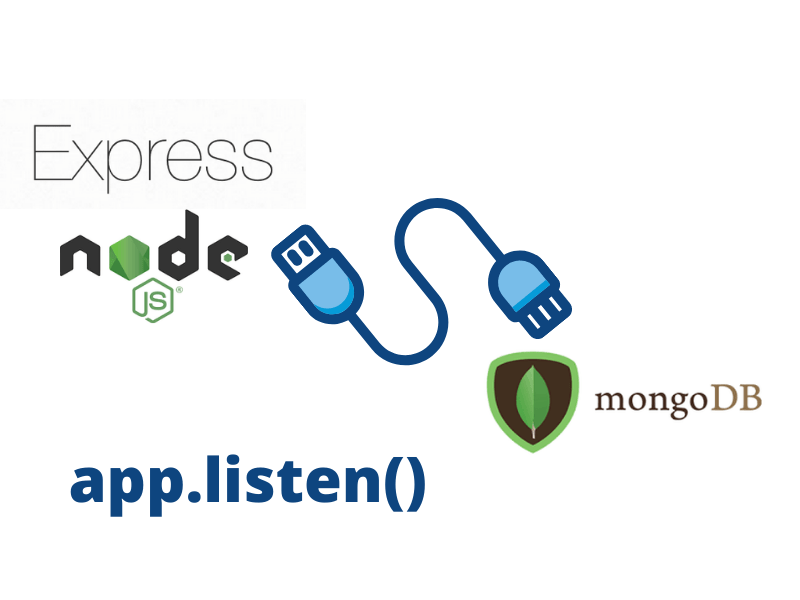
Starting an Express server and making the app listen is probably the first ever thing you did while learning Server-side JavaScript.
Its as easy as given here in Express docs.
Also, making a connection should not be that hard either. There are tons of tutorials out there. But, many tutorials out there, do not wait for a successful DB connection before the app startup.
For quite a few time, I was also doing the same. Make a DB connection and listen for HTTP connection in an asynchronous fashion, ignoring the state of the node app while the DB connection is being acquired.
While this might be not be bad for most of the cases, sometimes, the server is listening; would not be useful if the database connection is broken. In cases while our all routes need a DB connection, and we would not want the server running, if the database connection isn’t working. Then, in such a case, we can call app.listen in the DB connection callback.
App.listen in Mongoose Connect Callback
Here is what I meant. Here we are using Mongoose and while we have the connection with the DB, then we can call app.listen in the callback.
mongoose.connect('config.dbURL')
.then( (result) => {
app.listen(config.port, () => {
console.log(`Listening on port: ${config.port}`);
});
})
.catch( (err) => console.log('DB Connection Error: ', err.message))
Or, alternatively we can use Node’s EventEmitter and simply fire a ready event.
I am using VS Code while writing the snippets for the blog and its my favorite code editor. Which one is yours?
App.listen after DB connection using Node’s EventEmitter
We can use .once instance of Mongoose EventEmitter class, so that once our connection is open, we can we emit a ready event and listening for the event, we can make the app listen.
mongoose.connect('config.dbURL')
mongoose.connection.once('open',() => {
app.emit('ready');
});
app.on('ready', function() {
app.listen(config.port, () => {
console.log(`Listening on port: ${config.port}`);
});
});
In this way, our app is never in partially broken state.
A good explanation is given here.
Also, please take a look here.
While this might not be much of a big issue, just in case if all of your routes involve database communication, it might be useful.